Long running jobs
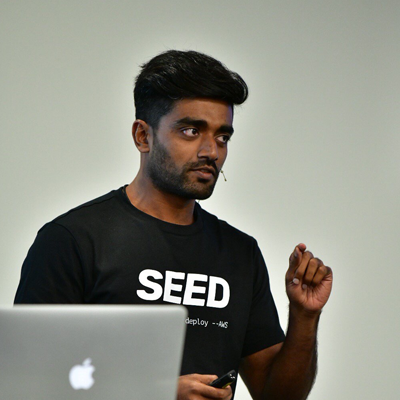
We launched a new construct that makes it easy to run functions longer than 15 minutes — Job
These are useful for cases where you are running async tasks like video processing, ETL, and ML. A Job
can run for up to 8 hours.
Job
is made up of:
Job
— a construct that creates the necessary infrastructure.JobHandler
— a handler function that wraps around your function code in a typesafe way.Job.run
— a helper function to invoke the job.
Launch event
We hosted a launch livestream where we demoed the new construct, did a deep dive, and answered some questions.
The video is timestamped and here’s roughly what we covered.
- Intro
- Demo
- Deep Dive
- Deep dive into the construct
- Granting permissions for running the job
- Typesafety
- Defining the job handler
- Running the job
- Live debugging the job
- Q&A
- Q: When should I use
Job
vsFunction
? - Q: Is
Job
a good fit for batch jobs? - Q: Why CodeBuild instead of Fargate?
- Q: When should I use
Get started
Here’s how you use the new Job
construct. Start by creating a new job.
import { Job } from "@serverless-stack/resources";
const job = new Job(stack, "MyJob", {
srcPath: "services",
handler: "functions/myJob.handler",
});
Add the job handler.
import { JobHandler } from "@serverless-stack/node/job";
declare module "@serverless-stack/node/job" {
export interface JobTypes {
MyJob: {
foo: string;
};
}
}
export const handler = JobHandler("MyJob", async (payload) => {
// Do the job
});
Finally invoke the job.
import { Job } from "@serverless-stack/node/job";
function someFunction() {
await Job.run("MyJob", {
payload: {
foo: "Hello World",
},
});
}
Note that the payload
and job name MyJob
here are typesafe.
For a full tutorial check out the Quick Start in the docs.